Since I started, I wobbled a number of times between a semantically messy 1980s style interpreted BASIC with line numbers and liberal use of GOTO/GOSUB and a slightly more modern version, block structuring, strict semantics, strong typing, but extensive type inference.
To me, BASIC will forever be:
10PRINT"HELLO WORLD"
20GOTO10
So, line numbers, no extraneous space and GOTO to handle branch control.
You could do scary stuff like this:
10GOSUB 100
20GOSUB 110
30END
100PRINT"HELLO ";
110PRINT"WORLD"
120RETURN
Two subroutines sharing a common RETURN statement!
So no line numbers then
So if you don’t have GOTO and GOSUB, you don’t need line numbers, and constructs like WHILE WEND / REPEAT UNTIL etc all work fine without line numbers.
But you also can’t just enter programs directly from booting up the computer – you need some kind of multi-line or full screen editor.
So, I committed to the latter and did indeed write a full screen editor.
Now, my SoapyBASIC looks like:
while true
greet
wend
sub greet()
print "Hello, world!"
endsub
Variables
Most early BASICs had predefined variables, sometimes only with one or two characters.
Numeric variables were just simple names like A
or C1
and string variables were terminated with a dollar sign, like X$
. Some later BASICs, used %
and !
suffices for integer and floating point numbers respectively.
I think BBC BASIC even went for %%
and !!
for long/double precision.
I didn’t want this. Also, I wanted to support single and double precision floating point, and all the signed and unsigned integers from 8 to 64-bits.
So my variable names attach no special meaning to the suffix and their types are inferred from declaration or can be explicitly stated.
Variables are introduced with let
like this:
let a=6 ' an integer
let b=2.5 ' a float
let c="Hello" ' a string
let d as uint8 = 55
' explicitly unsigned byte
After defining a variable you don’t use let
. You can’t use a variable before it’s been declared (and given a value) and it’s a type error to try to assign a value of the wrong type to a variable. These errors are caught before the program is run.
Arrays are mostly as expected, but you do have to specify the type when declaring, and I opted for square brackets for clarity. I know some old BASICs used square brackets, so not completely off.
dim x[10] of int
dim y[3] of string
' or use a literal
let z = [1,2,3] ' array of int
Loops
The classic for...next
loop is there, but for convenience I also have a for...in
loop for arrays. I also have while...wend
and repeat...until
for a=1 to 10:next
for b in [1,2,3]:next
let c=0
while c<10:c=c+1:wend
repeat:c=c-1:until c=0
User-defined subroutines and functions
Without goto
and gosub
I have user defined subroutines (statements that do not return a value) and multi-line and single-line user defined functions.
sub hello(p as string)
print "Hello, ";p
endsub
func twice(n as int) as int
return n+n
endfunc
def half(n as float) as float = n/2.0
hello "world"
print twice(9)
print half(7.2)
These definitions can be nested and recursion is fully supported.
A standard library
All the usual favourites are there except string functions do not end in a dollar sign.
So len
, mid
, chr
, asc
etc are there but as I fully support unicode, you also have code
to return a code point and other functions to break handle utf8.
There’s sin
, acos
, sqrt
etc.
The language itself does not support graphics, sound, etc, but supported modules will all have their own BASIC statements and functions.
The TTY version of SoapyBASIC has locate
, cls
, pen
, paper
, underline
, bright
etc.
Of course no BASIC is complete without the crazy syntax of print
and input
.
print "a";"b" ' ab
print "a","b" ' a b (tab)
print "abc"; ' do not print a new line
Finally, for now
I ended up with a language that still looks like BASIC to me, especially if you are familiar with newer dialects like QBASIC and BBC BASIC.
But it’s very strongly typed, warns you about most errors before the program is run. Has an editor that syntax highlights, and is relatively simple to interpret.
However, I’ve decided this year (2024) that I wanted to do it as a compiler. The host hardware is now fast enough to compile as you type, so that’s where I’m going next…
An example of SoapyBASIC
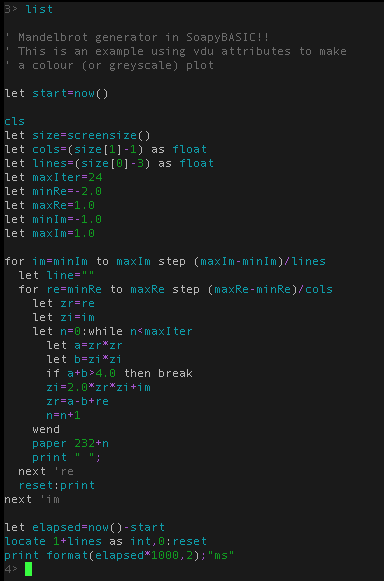
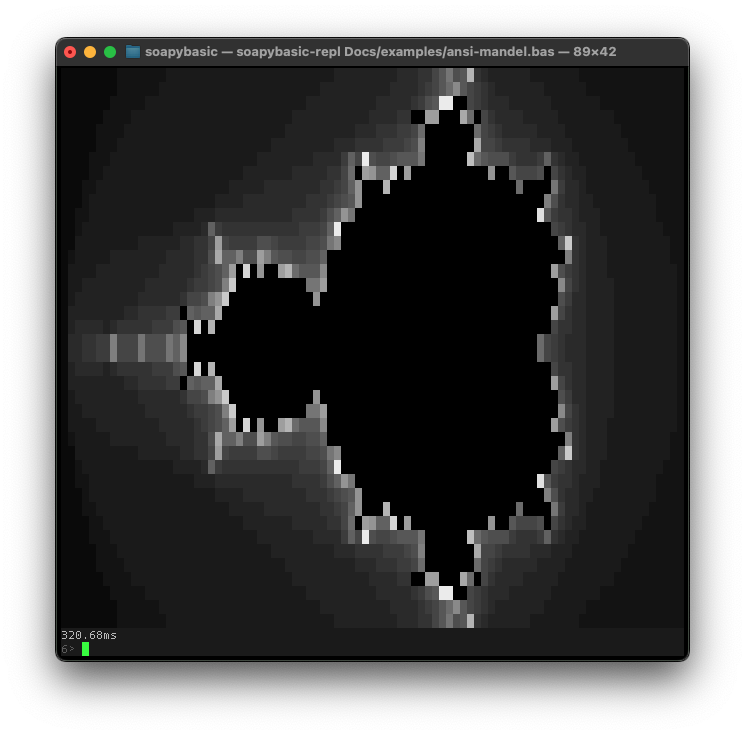
Leave a Reply